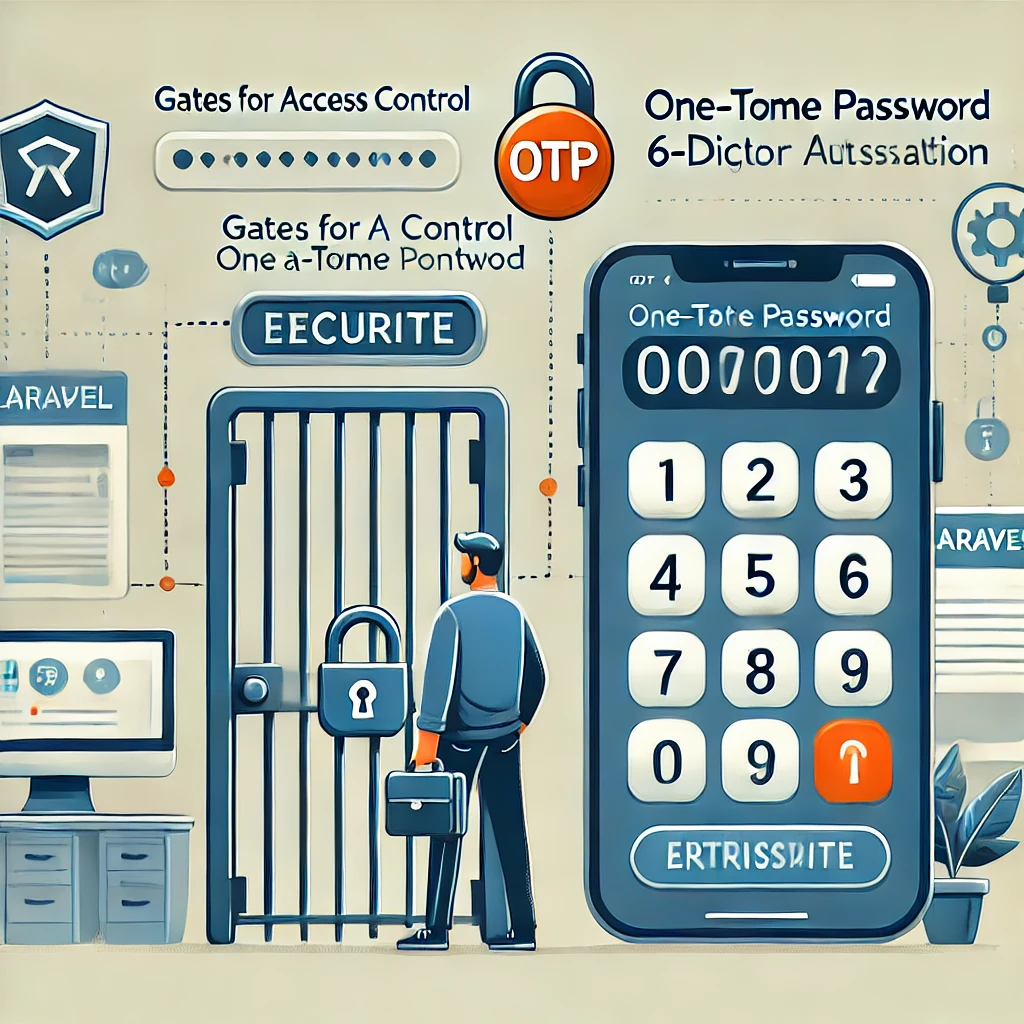
Security in Laravel and How to Strengthen It Using Gates and OTP
Security in Laravel and How to Strengthen It Using Gates and OTP
Laravel is one of the most popular frameworks for building modern web applications, and it comes with a variety of built-in security features to protect data and applications from various threats. In this article, we'll explore how to enhance security in Laravel using Gates and One-Time Passwords (OTP).
1. The Importance of Security in Web Applications
Data security and protection against threats like database attacks, fraud, and session manipulation are top priorities for every developer. A single security vulnerability can lead to a breach of sensitive data or even a full system compromise. Therefore, it's crucial to use best practices to strengthen security in Laravel applications.
2. Gates in Laravel
Gates in Laravel provide a simple way to authorize actions based on user roles or permissions. They serve as an access control mechanism, allowing you to define who can perform specific actions within the application.
How to Use Gates:
-
Defining the Gate: You can define a gate in the AuthServiceProvider. For example, to check if a user is an admin:
use Illuminate\Support\Facades\Gate; Gate::define('is-admin', function ($user) { return $user->role == 'admin'; });
-
Checking the Gate: To use the gate in any part of the application, you can call authorize:
if (Gate::allows('is-admin')) { // User is an admin } else { // Access denied }
You can also create other gates to verify permissions for different roles like "Content Editor" or "Regular User" using the same approach.
3. Enhancing Security with OTP (One-Time Password)
One-Time Passwords (OTP) are an effective way to enhance security by sending a code that is valid only for one use to verify a user's identity. OTPs are commonly used as part of Two-Factor Authentication (2FA), adding an extra layer of protection.
How to Add OTP in Laravel:
-
Installation and Setup: There are several packages that provide OTP support, such as Google Authenticator or Twilio for SMS-based OTP. In this example, we'll use the "laravel/fortify" package.
To install the package:
composer require laravel/fortify
-
Configuring Fortify: After installation, publish the configuration file:
php artisan vendor:publish --provider="Laravel\Fortify\FortifyServiceProvider"
-
Enabling 2FA: To enable two-factor authentication (2FA), you can add necessary fields to the users' table, such as
otp_code
andotp_expiry
, and send the OTP via email or SMS. -
Generating OTP: You can generate an OTP using packages like Google Authenticator or generate a random code using PHP:
$otp = rand(100000, 999999); // A 6-digit code
-
Verifying OTP: After sending the OTP to the user, you can verify the code:
if ($otp == $inputOtp) { // OTP is correct } else { // OTP is incorrect }
-
Sending OTP via Email or SMS: Using Twilio or Mail in Laravel, you can send OTP to the user via the preferred channels.
4. Best Practices for Enhancing Security in Laravel
-
Use HTTPS: Ensure that HTTPS is used for all web communications. This encrypts the data sent between the client and the server, preventing eavesdropping.
-
Security Updates: It is essential to stay up-to-date with regular security updates for the framework and any dependencies to patch any vulnerabilities.
-
Input Validation: Always use input sanitization and validation to prevent attacks like SQL Injection and Cross-site Scripting (XSS).
-
Two-Factor Authentication (2FA): Enabling two-factor authentication using OTP adds a significant layer of security, ensuring that even if someone has the password, they can't access the account without the OTP.
-
Precise Access Control with Gates: Use Gates to precisely check user permissions. Make sure that every sensitive action within the application requires a valid authorization check.
5. Lastly but not least
Enhancing security in Laravel applications is a necessary step to protect user data and prevent emerging threats. By using features like Gates for access control and OTP for two-factor authentication, you can significantly increase the security of your application. Adhering to best security practices will ensure that your web applications are more secure and reliable.